Raycast Unity 2D Step by step guide
When building a 2D game in Unity, one of the most essential techniques for player interaction, AI detection, and shooting mechanics is raycasting. Whether you’re creating a top-down shooter, a puzzle platformer, or a stealth-based adventure, learning how to implement 2D Raycast in Unity gives you precise control over object detection and game logic.
In this tutorial, we’ll explore how to use Raycast Unity 2D to detect objects, trigger interactions, and implement features like line-of-sight and projectile hits. You’ll learn how to work with Physics2D Raycast, how to use RaycastHit2D Unity for hit detection, and even how to raycast from camera in Unity for mouse or touch input.

We’ll cover everything from basic Ray2D Unity usage to more advanced applications like shooting mechanics and interactive elements. Whether you’re new to Unity 2D Raycast or looking to level up your current implementation, this guide will help you master Unity Physics2D Raycast like a pro.
Let’s dive in and unlock the full power of Raycasting in Unity 2D!
Understanding Raycast in Unity 2D

A. Definition and basic concept
Raycast in Unity 2D is a powerful tool for detecting objects and interactions within a 2D game environment. It works by casting an invisible line (ray) from a specified origin point in a particular direction, allowing developers to check for collisions with objects along that line. This technique is essential for implementing various gameplay mechanics, such as shooting, object detection, and character interactions.
B. Advantages of using Raycast in 2D games
Using Raycast in 2D games offers several benefits:
- Efficient object detection
- Precise collision detection
- Versatile interaction implementation
- Performance optimization
Advantage | Description |
---|---|
Efficiency | Raycast performs quick checks without physically moving objects |
Precision | Allows for accurate hit detection and distance calculations |
Versatility | Can be used for various gameplay mechanics and interactions |
Optimization | Reduces the need for constant collision checks between objects |
C. Key components of Raycast in Unity 2D
The main components of Raycast in Unity 2D include:
- Origin point: The starting position of the ray
- Direction: The vector indicating the ray’s trajectory
- Distance: The maximum length of the ray
- LayerMask: Determines which layers the ray can interact with
- RaycastHit2D: Stores information about detected collisions
Understanding these components is crucial for effectively implementing Raycast in Unity 2D projects. By mastering Raycast, developers can create more interactive and dynamic 2D game environments. Next, we’ll explore how to set up 2D Raycast for object detection in Unity.
Setting Up 2D Raycast for Object Detection

Configuring LayerMasks for specific object targeting
LayerMasks are essential for efficient object detection in Unity 2D Raycast. They allow you to filter which objects the raycast should interact with, improving performance and precision.
To set up a LayerMask:
- Create layers for different object types in Unity’s Layer Manager
- Assign objects to appropriate layers in the Inspector
- Define a LayerMask variable in your script
- Use bitwise operations to include or exclude specific layers
Here’s an example of configuring a LayerMask:
public LayerMask targetLayers;
void Start()
{
targetLayers = LayerMask.GetMask("Enemy", "Obstacle");
}
Implementing Raycast origin and direction
The origin and direction of a raycast determine its starting point and the path it follows. In 2D, you’ll typically use Vector2 for both.
Key points for implementation:
- Origin: Usually the position of the object casting the ray
- Direction: Normalized vector pointing in the desired direction
Example code:
Vector2 origin = transform.position;
Vector2 direction = transform.right; // Assumes object is facing right
Handling Raycast 2D hit information
When a raycast hits an object, it returns a RaycastHit2D struct containing valuable information about the collision. Here’s how to handle it:
- Perform the raycast using Physics2D.Raycast()
- Check if the raycast hit anything
- Access hit information like point of contact, distance, and collider
Example:
RaycastHit2D hit = Physics2D.Raycast(origin, direction, maxDistance, targetLayers);
if (hit.collider != null)
{
Debug.Log("Hit object: " + hit.collider.gameObject.name);
Debug.Log("Hit point: " + hit.point);
Debug.Log("Hit distance: " + hit.distance);
}
Visualizing Raycasts for debugging
Visualizing raycasts is crucial for debugging and ensuring proper implementation. Unity provides tools to draw lines representing raycasts in the Scene view and Game view.
Methods for visualization:
- Debug.DrawRay(): Draws a ray from a start point in a direction
- Debug.DrawLine(): Draws a line between two points
Example usage:
void Update()
{
Vector2 origin = transform.position;
Vector2 direction = transform.right;
float maxDistance = 10f;
RaycastHit2D hit = Physics2D.Raycast(origin, direction, maxDistance, targetLayers);
if (hit.collider != null)
(origin, hit.point, Color.red);
}
else
{
Debug.DrawRay(origin, direction * maxDistance, Color.green);
}
}
This code visualizes the raycast in red when it hits something and green when it doesn’t, making it easy to see its behavior in the Unity editor.
Implementing Shooting Mechanics with raycast 2d unity
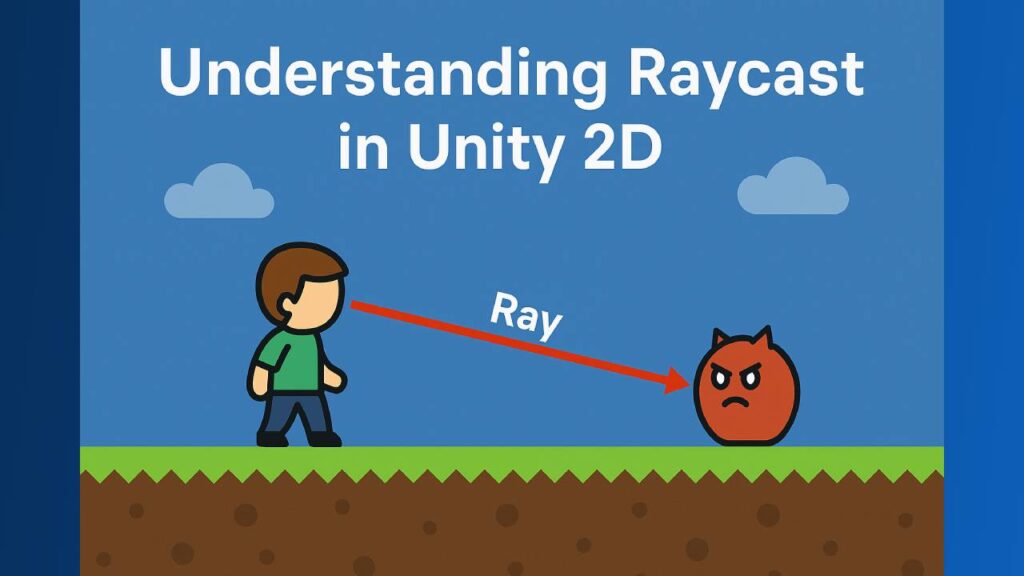
Creating a basic shooting system
To implement a basic shooting system in Unity 2D using Raycast, we’ll start by setting up a player character and a shooting mechanism. Here’s a step-by-step approach:
- Create a player GameObject
- Attach a script for player movement
- Add a shooting point (empty GameObject) as a child of the player
- Implement the shooting logic using Raycast2D
public class PlayerShooting : MonoBehaviour
{
public Transform shootingPoint;
public float shootingRange = 10f;
public LayerMask targetLayer;
void Update()
{
if (Input.GetButtonDown("Fire1"))
{
Shoot();
}
}
void Shoot()
{
RaycastHit2D hit = Physics2D.Raycast(shootingPoint.position, shootingPoint.right, shootingRange, targetLayer);
if (hit.collider != null)
{
Debug.Log("Hit: " + hit.collider.name);
}
}
}
Calculating bullet trajectory using Raycast 2D
Raycast2D allows us to simulate bullet trajectories accurately. We can use it to determine the path and destination of our projectiles:
- Calculate the direction vector
- Use Physics2D.Raycast to detect collisions
- Visualize the trajectory using Debug.DrawRay
Parameter | Description |
---|---|
Origin | Starting point of the ray |
Direction | Direction of the ray |
Distance | Maximum distance of the ray |
LayerMask | Layers to include in collision detection |
Applying damage to detected objects
Once we’ve detected a hit using Raycast2D, we can apply damage to the target:
- Create an interface for damageable objects
- Implement the interface in target scripts
- Apply damage when a hit is detected
public interface IDamageable
{
void TakeDamage(float damage);
}
// In PlayerShooting script
void Shoot()
{
RaycastHit2D hit = Physics2D.Raycast(shootingPoint.position, shootingPoint.right, shootingRange, targetLayer);
if (hit.collider != null)
{
IDamageable damageable = hit.collider.GetComponent<IDamageable>();
if (damageable != null)
{
damageable.TakeDamage(10f);
}
}
}
Adding visual effects for shooting
To enhance the shooting experience, we can add visual effects:
- Create a line renderer for bullet trails
- Instantiate particle effects at impact points
- Add screen shake for a more dynamic feel
These effects will make the shooting mechanic more engaging and provide better feedback to the player. In the next section, we’ll explore how to use Raycast for enhancing interaction in your Unity 2D game.
Enhancing Interaction Using 2d raycast unity

Detecting interactive objects in the game world
In Unity 2D, Raycast provides an efficient way to detect interactive objects in your game world. By using Physics2D.Raycast, you can send out a ray from a specific point in a defined direction to identify objects with colliders.
Here’s a simple example of how to detect interactive objects:
RaycastHit2D hit = Physics2D.Raycast(transform.position, Vector2.right, 5f);
if (hit.collider != null && hit.collider.CompareTag("Interactive"))
{
Debug.Log("Interactive object detected: " + hit.collider.gameObject.name);
}
Implementing context-sensitive actions
Once you’ve detected an interactive object, you can implement context-sensitive actions based on the object’s properties. This enhances the player’s experience by providing dynamic interactions.
Object Type | Action |
---|---|
Door | Open/Close |
Item | Pick up |
NPC | Start dialogue |
Switch | Toggle on/off |
Here’s how you might implement this:
void HandleInteraction(RaycastHit2D hit)
{
switch (hit.collider.gameObject.tag)
{
case "Door":
OpenDoor(hit.collider.gameObject);
break;
case "Item":
PickUpItem(hit.collider.gameObject);
break;
// Add more cases as needed
}
}
Creating a highlight system for interactable objects
To improve user experience, implement a highlight system for interactable objects. This visual feedback helps players identify which objects they can interact with.
- Create a shader for highlighting
- Apply the shader to interactable objects
- Toggle the highlight effect when the raycast detects an object
Here’s a basic script to toggle highlighting:
void Update()
{
RaycastHit2D hit = Physics2D.Raycast(transform.position, Vector2.right, 5f);
if (hit.collider != null && hit.collider.CompareTag("Interactive"))
{
HighlightObject(hit.collider.gameObject, true);
}
else
ighlight all objects
HighlightObject(null, false);
}
}
void HighlightObject(GameObject obj, bool highlight)
{
// Apply or remove highlight shader
}
By implementing these techniques, you’ll create a more engaging and intuitive interaction system in your Unity 2D game. Next, we’ll explore how to optimize 2D Raycast performance to ensure smooth gameplay even with complex interaction systems.
Optimizing 2D Raycast Performance

Limiting Raycast frequency
To optimize 2D Raycast performance in Unity, it’s crucial to limit the frequency of Raycast calls. Excessive Raycasting can significantly impact your game’s performance. Here are some strategies to reduce Raycast frequency:
- Use coroutines for periodic Raycasting
- Implement cooldown timers
- Raycast only when necessary (e.g., on player input)
| Method | Pros | Cons |
|-----------------------|----------------------------------|------------------------------------|
| Coroutines | Smooth performance, customizable | Slightly more complex to implement |
| Cooldown timers | Simple to implement | Less flexible |
| On-demand Raycasting | Minimal performance impact | May miss some detections |
Using efficient collision detection techniques
Combine Raycasting with other collision detection methods for optimal performance:
- Use colliders for initial broad-phase detection
- Apply Raycasting for precise hit detection
- Utilize Unity’s built-in 2D physics system efficiently
Balancing accuracy and performance
Finding the right balance between accuracy and performance is key:
- Adjust Raycast length: Shorter rays are more efficient
- Optimize layer masks: Target only relevant layers
- Use RaycastAll sparingly: It’s more expensive than single Raycasts
Remember, the goal is to maintain smooth gameplay while ensuring accurate object detection and interaction. Experiment with these techniques to find the optimal solution for your Unity 2D project.
Advanced Unity 2D Raycast Techniques

Multiple Raycasts for area detection
When dealing with complex scenarios in Unity 2D, using multiple raycasts can significantly enhance your object detection capabilities. This technique allows you to create a fan-shaped detection area or simulate a wider field of view.
To implement multiple raycasts:
- Define the number of rays
- Calculate the angle between each ray
- Cast rays in a loop, adjusting the angle for each
Here’s a sample code snippet:
public int rayCount = 5;
public float rayAngle = 45f;
void CastMultipleRays()
{
float angleStep = rayAngle / (rayCount - 1);
for (int i = 0; i < rayCount; i++)
{
float angle = -rayAngle / 2 + angleStep * i;
Vector2 direction = Quaternion.Euler(0, 0, angle) * transform.right;
RaycastHit2D hit = Physics2D.Raycast(transform.position, direction, detectionRange);
// Process hit results
}
}
Implementing 2D line of sight
Creating a line of sight system in 2D involves using raycasts to determine visibility between two points. This technique is crucial for stealth games or AI vision systems.
Steps to implement 2D line of sight:
- Cast a ray from the observer to the target
- Check for obstacles using layer masks
- Determine if the target is visible based on hit results
Component | Purpose |
---|---|
Observer | Starting point of the raycast |
Target | End point of the raycast |
Obstacles | Objects that block line of sight |
Layer Mask | Filters which objects to consider |
Creating realistic ricochet effects
Raycasts can be used to create realistic ricochet effects for projectiles in 2D games. This technique involves calculating the reflection angle based on the surface normal of the hit object.
To implement ricochet effects:
- Cast a ray in the projectile’s direction
- If a collision occurs, calculate the reflection vector
- Spawn a new projectile or change the current one’s direction
Using Raycast for AI behavior and pathfinding
Raycasts are invaluable for implementing AI behavior and pathfinding in 2D games. They can be used to detect obstacles, find targets, and make decisions based on the environment.
Key applications of raycasts in AI:
- Obstacle avoidance
- Target detection
- Wall following
- Simple pathfinding
By combining these advanced techniques, you can create more sophisticated and engaging 2D games using Unity’s raycast system. These methods provide a solid foundation for implementing complex game mechanics and AI behaviors.
Raycasting from camera to screen in unity
Understanding Screen Point to Ray Conversion
In Unity, converting screen points to rays is crucial for camera-based raycasting. This process involves transforming 2D screen coordinates into a 3D ray in the game world.
Key Concepts:
- Screen coordinates: (0,0) at bottom-left, (Screen.width, Screen.height) at top-right
- Viewport coordinates: Normalized (0,0) to (1,1)
- World coordinates: 3D space in your game world
Coordinate System | Range | Origin |
---|---|---|
Screen | (0,0) to (width,height) | Bottom-left |
Viewport | (0,0) to (1,1) | Bottom-left |
World | 3D space | Scene-dependent |
Raycasting from Mouse Position to Game World
To raycast from the mouse position:
- Get mouse position in screen coordinates
- Convert to viewport or world coordinates
- Create a ray from the camera through this point
Vector3 mousePos = Input.mousePosition;
Ray ray = Camera.main.ScreenPointToRay(mousePos);
Detecting Objects with Camera-Based Raycast
Once you have the ray, use Physics2D.Raycast to detect objects:
- Set appropriate layer masks
- Use RaycastHit2D to store hit information
Code Example: Raycasting from Camera on Mouse Click
void Update()
{
if (Input.GetMouseButtonDown(0))
{
Ray ray = Camera.main.ScreenPointToRay(Input.mousePosition);
RaycastHit2D hit = Physics2D.Raycast(ray.origin, ray.direction);
if (hit.collider != null)
{
Debug.Log("Hit object: " + hit.collider.gameObject.name);
}
}
}
Common Pitfalls and How to Fix Them
- Wrong camera: Ensure you’re using the correct camera for raycasting
- Layer masks: Use appropriate layer masks to filter objects
- 2D vs 3D: Remember to use Physics2D for 2D projects
- Z-depth: Consider z-depth when working with 2D objects in a 3D space
Now that we’ve covered camera-based raycasting, let’s explore some advanced Unity 2D Raycast techniques to further enhance your game development skills.
Unity Raycast alternatives
Also Check:
- first unity game
- game development tips
- Implement Multiplayer in Unity
- game on google play store
- Unity Engine Asset Store
Unity Raycast 2D Alternatives
A. Using Physics.Overlap Methods for Area-Based Detection
Physics.Overlap methods provide an efficient way to detect objects within a specific area. These methods are particularly useful when you need to check for multiple objects simultaneously.
Method | Description | Use Case |
---|---|---|
Physics2D.OverlapCircle | Detects colliders within a circular area | Explosion radius, pickup detection |
Physics2D.OverlapBox | Detects colliders within a rectangular area | Area of effect abilities, trigger zones |
Physics2D.OverlapArea | Detects colliders within an arbitrary area | Custom-shaped detection zones |
B. Linecasting in Unity: A Simpler Raycast Alternative
Linecasting is a simplified version of raycasting that checks for collisions along a line segment. It’s ideal for quick, short-range detections.
- Use Physics2D.Linecast for 2D applications
- Efficient for simple collision checks
- Useful for character ground checks or short-range interactions
C. Using Trigger Colliders for Continuous Detection
Trigger colliders offer a passive approach to object detection:
- Attach a trigger collider to your object
- Implement OnTriggerEnter2D, OnTriggerStay2D, and OnTriggerExit2D methods
- Detect and interact with objects that enter the trigger zone
This method is excellent for continuous detection without the need for constant raycasting.
D. Grid-Based Detection for Tile-Based Games
For tile-based games, a grid system can replace raycasting:
- Divide your game world into a grid
- Store object positions in grid cells
- Use simple coordinate checks for detection and interaction
This approach is highly efficient for games with discrete movement or placement.
Conclusion – Raycast hit 2d unity
Mastering 2D Raycast in Unity is a game-changer for developing interactive, responsive, and dynamic gameplay. Whether you’re detecting enemy collisions, firing projectiles, or allowing your player to interact with the environment, Unity 2D Raycast and Physics2D Raycast give you the precision and control you need.
By using RaycastHit2D Unity effectively, you can retrieve valuable information about what your ray hits and respond accordingly—be it triggering an event, damaging an enemy, or selecting an object. And if you’re building input-driven mechanics, combining Raycast from Camera Unity with Physics2D.Raycast is perfect for translating user input into in-game actions.
From basic Ray2D Unity usage to advanced shooting mechanics, you’ve now got the tools and knowledge to enhance your game’s interactivity and logic. Keep experimenting with Unity RaycastHit2D and continue exploring what’s possible with Raycast Unity 2D—your gameplay will be sharper, smarter, and more immersive than ever before.
FAQ on Unity Raycast 2D
1. What is a Raycast in Unity 2D?
A Raycast in Unity 2D is a way to detect objects in a straight line from a specific point in a 2D space. It uses Physics2D.Raycast
to check if any colliders intersect with the ray, which is useful for mechanics like shooting, interaction, and enemy detection.
2. How do I use Physics2D.Raycast in a Unity 2D project?
You can use Physics2D.Raycast(origin, direction)
in your script to cast a ray from one point in a specific direction. It returns a RaycastHit2D
object that contains details about what the ray hit, including the collider, distance, and impact point.
3. Can I Raycast from the camera in Unity 2D?
Yes, you can use Camera.main.ScreenToWorldPoint()
to convert screen coordinates (like mouse position) to world space, and then cast a 2D ray from that point. This is useful for selecting objects with mouse or touch input in 2D games.
4. What’s the difference between Raycast and Linecast in Unity?
Both are similar, but Raycast
shoots a ray in a direction, while Linecast
checks along a defined line segment between two points. Linecast
is often used for checking direct visibility or obstacles between objects in 2D.
5. How can I optimize 2D Raycast performance?
To optimize Unity 2D Raycasts, limit the raycast distance, use layer masks to ignore irrelevant objects, and avoid excessive raycasting every frame if not necessary. Reuse logic or cache results when possible.
Happy coding, and may your rays always hit their mark! 🎯🚀