Building a multiplayer game in Unity requires the right approach to ensure smooth connectivity and performance. Choosing the best multiplayer framework for Unity depends on your game’s requirements, whether it’s a co-op, competitive, or MMO experience. A proper Unity networking setup includes selecting a framework like Netcode for GameObjects, Photon, Mirror, setting up synchronization, and optimizing performance.
A solid Unity multiplayer implementation prevents lag and ensures a seamless experience for players. This guide covers everything you need to know about implementing multiplayer in Unity, from setup to debugging, so you can create a great online game. 🚀
Unity multiplayer implementation – Step by Step guide
Embarking on a Unity multiplayer implementation? 🎮 Want to learn how to implement multiplayer in unity? the path to creating an engaging multiplayer experience can be as thrilling as it is daunting. With a myriad of networking options and technical challenges, developers often find themselves at a crossroads, unsure of the best approach to bring their multiplayer vision to life.
Unity offers a robust platform for multiplayer game development, but choosing the right implementation method is crucial for success in unity game development. From selecting the appropriate networking framework to optimizing performance across various network conditions, each decision can significantly impact the final product. This guide will navigate you through the intricacies of multiplayer implementation in Unity, helping you avoid common pitfalls and leverage the platform’s full potential.
As we delve into the best practices for implementing multiplayer in Unity, we’ll explore everything from understanding Unity’s multiplayer options to testing and debugging your networked game. Whether you’re a seasoned developer or just starting out, this comprehensive walkthrough will equip you with the knowledge to create seamless, engaging multiplayer experiences that keep players coming back for more.

Understanding Unity’s Multiplayer Options
When exploring Unity’s multiplayer options, it is crucial to grasp the intricacies and best practices to ensure a seamless gaming experience. By understanding Unity’s various networking capabilities and optimal performance strategies, developers can create engaging and stable multiplayer games. Testing and debugging networked games is also essential to identify and address potential issues early on. This guide provides comprehensive insights into Unity’s multiplayer features, offering valuable knowledge for developers at all levels.
Unity Networking (UNET)
Unity Networking (UNET) was Unity’s built-in networking solution for multiplayer game development. Although it has been deprecated, understanding its principles can provide valuable insights into Unity’s approach to multiplayer implementation.
UNET offered a high-level API for rapid multiplayer prototyping and a low-level API for more advanced network programming. It provided features such as network transforms, client-side prediction, and a lobby system. However, due to its limitations and the evolving needs of game developers, Unity has since moved on to more modern networking solutions.
Mirror Networking
Mirror is an open-source networking solution that emerged as a popular alternative to UNET. It’s designed to be a drop-in replacement for UNET, making it easier for developers familiar with UNET to transition to a more actively maintained framework.
Key features of Mirror include:
- Easy-to-use high-level API
- Efficient network synchronization
- Compatibility with Unity’s networking components
- Active community support and regular updates
Mirror’s simplicity and familiarity make it an excellent choice for developers looking to implement multiplayer functionality in Unity quickly.
Photon Networking
Photon is a third-party networking solution that offers both free and paid plans, making it suitable for projects of various scales. It provides a robust and scalable infrastructure for multiplayer games, handling many of the complex networking tasks for developers.
Photon offers several products:
- Photon PUN (Photon Unity Networking)
- Photon Realtime
- Photon Quantum
Each product caters to different multiplayer needs, from simple turn-based games to complex real-time multiplayer experiences.
Custom Solutions
For projects with specific requirements or performance demands, custom networking solutions can be developed using low-level networking protocols. This approach offers maximum flexibility but requires more development time and expertise.
Networking Solution | Pros | Cons |
---|---|---|
UNET (Deprecated) | – Integrated with Unity- Familiar to many developers | – No longer supported- Limited scalability |
Mirror | – Open-source- Similar to UNET- Active community | – May require additional setup- Less extensive documentation |
Photon | – Robust infrastructure- Scalable- Multiple products | – Potential costs for larger projects- Less control over network stack |
Custom Solutions | – Full control- Tailored to specific needs | – Time-consuming- Requires networking expertise |
When selecting a multiplayer networking solution for Unity, consider factors such as project scale, performance requirements, development timeline, and team expertise. Each option offers unique advantages and trade-offs, making it essential to align the choice with the specific needs of the game project.
By understanding these Unity multiplayer options, developers can make informed decisions on the best approach for implementing multiplayer functionality in their games. The choice of networking solution will significantly impact the development process and the final product’s performance and scalability.
Choosing the Right Networking Framework
Selecting the right networking framework is crucial for implementing multiplayer in Unity. The best choice depends on your game’s scale, performance needs, and budget. Unity offers Netcode for GameObjects, ideal for small to mid-sized games. Photon (PUN & Fusion) provides a cloud-based solution for real-time action games. Mirror is a great open-source alternative for server-authoritative multiplayer. Each framework has its strengths and weaknesses, so understanding their capabilities will help you create a smooth and scalable multiplayer experience. Let’s explore which option best fits your project’s needs. 🚀
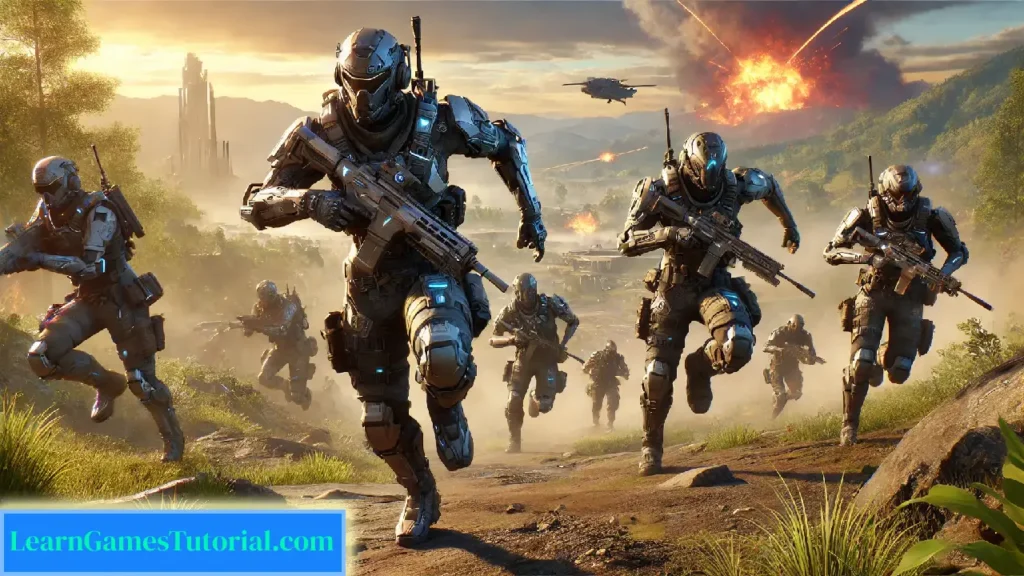
Read More – Photon vs. Netcode Comparision: Which Networking Solution is Best?
Assessing Project Requirements
When selecting a networking framework for your Unity multiplayer project, it’s crucial to start by thoroughly assessing your project requirements. This step ensures that you choose a solution that aligns with your game’s specific needs and technical constraints.
Consider the following factors:
- Game Genre: Different game types have varying networking demands. For instance, a fast-paced first-person shooter requires low latency and frequent updates, while a turn-based strategy game can tolerate higher latency.
- Player Count: Determine the maximum number of players your game will support simultaneously. This affects the choice of networking architecture and server infrastructure.
- Platform Support: Ensure the framework supports all platforms you intend to release on, including mobile, desktop, and consoles.
- Real-time vs. Turn-based: Real-time games require more frequent state updates and synchronization, while turn-based games can use simpler networking models.
- Gameplay Features: Consider specific features like physics simulations, AI behavior, or persistent world states that may influence your networking needs.
Scalability Considerations
Scalability is a critical factor in choosing a networking framework, especially if you anticipate your game growing in popularity or complexity over time.
Key scalability aspects to consider:
- Player Capacity: Evaluate how well the framework handles an increasing number of concurrent players.
- Server Architecture: Consider whether a peer-to-peer, client-server, or hybrid architecture best suits your scalability needs.
- Cloud Integration: Look for frameworks that offer seamless integration with cloud services for easier scaling of server resources.
- Load Balancing: Assess the framework’s ability to distribute network traffic efficiently across multiple servers.
Here’s a comparison of popular Unity networking frameworks in terms of scalability:
Framework | Max Players | Architecture | Cloud Integration | Load Balancing |
---|---|---|---|---|
Photon | 100,000+ | Client-Server | Yes | Yes |
Mirror | Unlimited | Flexible | Manual | Manual |
Unity Networking | 100+ | Client-Server | Limited | Limited |
Fish-Net | Unlimited | Flexible | Manual | Manual |
Ease of Implementation
The ease of implementation can significantly impact development time and resource allocation. Consider these factors:
- Learning Curve: Assess the time and effort required to learn and integrate the framework into your project.
- Documentation: Look for frameworks with comprehensive, up-to-date documentation and active community support.
- Integration with Unity: Choose frameworks that seamlessly integrate with Unity’s workflow and component system.
- Code Complexity: Evaluate the amount of boilerplate code required and the overall complexity of implementing common networking tasks.
Cost Factors
Finally, consider the cost implications of your chosen networking framework:
- Licensing Fees: Compare the pricing models of different frameworks, including free, one-time purchase, or subscription-based options.
- Server Hosting Costs: Estimate the expenses associated with hosting game servers, whether on-premises or cloud-based.
- Development Time: Factor in the potential costs of extended development time due to complex implementation or learning curves.
- Scalability Costs: Consider how costs may increase as your player base grows and server requirements expand.
Now that we’ve covered the key aspects of choosing the right networking framework, let’s explore how to set up the multiplayer environment using your selected solution.
Setting Up the Multiplayer Environment
To set up a multiplayer environment in Unity, start by selecting a networking framework like Netcode for GameObjects, Photon, or Mirror. Configure network managers, player spawning, and synchronization. Use Unity Relay & Lobby for matchmaking or set up a dedicated server for better performance and scalability. 🚀
Creating a Multiplayer Scene
When setting up a multiplayer environment in Unity, the first step is creating a dedicated multiplayer scene. This scene serves as the foundation for your networked gameplay. Begin by designing a layout that accommodates multiple players, considering spawn points, shared resources, and interactive elements.

Ensure your scene includes:
- Player spawn points: Strategically place empty GameObjects to mark where players will enter the game.
- Networked objects: Identify which objects in the scene need to be synchronized across the network.
- UI elements: Create a user interface for lobby management, player status, and game-specific information.
Remember to optimize your scene for network performance by reducing unnecessary visual elements and using efficient lighting techniques.
Implementing Player Prefabs
Player prefabs are essential components in Unity multiplayer games. They represent the networked player objects that will be instantiated for each connected client. When creating player prefabs:
- Design a modular player model with separate components for visuals, controls, and network behavior.
- Attach a NetworkIdentity component to the prefab to enable network synchronization.
- Implement NetworkTransform for smooth position and rotation updates.
- Add custom scripts for player-specific logic and input handling.
Here’s a comparison of key components in a typical player prefab:
Component | Purpose | Network Relevance |
---|---|---|
NetworkIdentity | Uniquely identifies the player on the network | Essential |
NetworkTransform | Synchronizes position and rotation | High |
PlayerController | Handles player input and movement | Local execution, network sync |
PlayerStats | Manages player health, score, etc. | Requires synchronization |
PlayerVisuals | Controls player appearance | Optional network sync |
Configuring Network Manager
The Network Manager is a crucial component that oversees the multiplayer functionality in Unity. To configure it effectively:
- Add the Network Manager component to an empty GameObject in your scene.
- Set up the Network Manager HUD for easy testing and debugging.
- Assign your player prefab to the “Player Prefab” field in the Network Manager.
- Configure network settings such as maximum connections, scene management, and spawn positions.
Fine-tune the Network Manager’s advanced settings to optimize performance and handle disconnections gracefully. Consider implementing custom spawn management for more complex gameplay scenarios.
Establishing Server-Client Architecture
Implementing a robust server-client architecture is crucial for a stable multiplayer experience. Unity’s networking system typically follows a client-authoritative model with server validation. To establish this architecture:
- Determine the hosting method: dedicated server, listen server, or peer-to-peer.
- Implement server-side logic for game state management and client request validation.
- Design a client-side prediction and reconciliation system to handle network latency.
- Create a reliable communication protocol for important game events and state updates.
Consider using Unity’s built-in networking commands like [Command] for client-to-server communication and [ClientRpc] for server-to-client updates. Implement proper security measures to prevent cheating and ensure fair gameplay across all connected clients.
Synchronizing your Multiplayer Game State
Synchronizing game state ensures all players experience a consistent world. This involves syncing player movements, animations, object interactions, and game events across the network. Networking frameworks like Netcode for Game Objects, Photon, and Mirror offer built-in solutions for state synchronization. Techniques like client prediction, server reconciliation, and interpolation help reduce lag and ensure smooth gameplay. To optimize performance, only essential data should be transmitted, using data compression and delta updates. Implementing server authority can prevent cheating and ensure fair gameplay. Proper synchronization is key to delivering a seamless and immersive multiplayer experience in Unity.
Network Transform Components
Network Transform components are essential tools in Unity for synchronizing the position, rotation, and scale of game objects across the network. These components automatically handle the replication of transform data, ensuring that all connected clients see objects in the same position and orientation.
To implement Network Transform components effectively:
- Add the Network Transform component to any game object that needs to be synchronized across the network.
- Configure the component’s settings, such as update frequency and interpolation, to balance between smooth movement and network efficiency.
- Use the Network Transform Child component for objects that are parented to other networked objects.
Custom Network Behaviors
While Network Transform components handle basic synchronization, custom network behaviors allow for more complex and game-specific synchronization logic. These behaviors are implemented using Unity’s networking API and can be tailored to your game’s specific needs.
To create custom network behaviors:
- Inherit from the NetworkBehaviour class when creating scripts for networked objects.
- Use [SyncVar] attribute to automatically synchronize variables across the network.
- Implement custom serialization methods for complex data structures that need synchronization.
Remote Procedure Calls (RPCs)
Remote Procedure Calls (RPCs) are a powerful feature in Unity’s networking system that allows you to execute functions on remote clients or the server. RPCs are crucial for implementing actions that need to be triggered across the network, such as player actions or game events.
Types of RPCs in Unity:
RPC Type | Description | Use Case |
---|---|---|
Server RPC | Called by clients, executed on the server | Player actions, game state updates |
Client RPC | Called by the server, executed on all clients | Visual effects, UI updates |
TargetRPC | Called by the server, executed on a specific client | Player-specific notifications |
To use RPCs effectively:
- Mark methods with appropriate RPC attributes ([ServerRpc], [ClientRpc], or [TargetRpc]).
- Ensure that RPCs are called from the correct context (client or server).
- Use RPCs judiciously to avoid overwhelming the network with frequent calls.
State Synchronization Techniques
Efficient state synchronization is crucial for maintaining a consistent game state across all connected clients. Various techniques can be employed to optimize this process:
- Delta Compression: Only send changes in state rather than the entire state.
- Interpolation and Extrapolation: Smooth out movement between network updates.
- Prediction and Reconciliation: Predict future states on the client and reconcile with server updates.
Implementing these techniques requires careful consideration of your game’s specific requirements and network conditions. It’s essential to strike a balance between responsiveness and network efficiency.
With these synchronization methods in place, your Unity multiplayer game will maintain a consistent state across all connected clients, providing a smooth and enjoyable multiplayer experience. Next, we’ll explore how to optimize network performance to further enhance the multiplayer gameplay.
Optimizing Network Performance of Multiplayer game

Reducing Bandwidth Usage
Efficient bandwidth management is crucial for a smooth multiplayer experience in Unity. To reduce bandwidth usage, consider implementing the following strategies:
- Data Compression: Utilize compression algorithms to minimize the size of data packets sent over the network.
- Delta Compression: Send only the changes in game state rather than the entire state each update.
- Prioritization: Transmit critical data more frequently than less important information.
- Culling: Limit the amount of data sent to each client based on relevance and visibility.
Implementing Prediction and Interpolation
Prediction and interpolation techniques can significantly improve the perceived responsiveness of multiplayer games:
- Client-Side Prediction: Allow the client to predict and execute actions locally before receiving server confirmation.
- Server Reconciliation: Correct client predictions when server updates arrive to maintain consistency.
- Entity Interpolation: Smoothly interpolate object positions between network updates to reduce visual jitter.
Handling Latency Issues
Latency can severely impact player experience. Implement these techniques to mitigate its effects:
- Lag Compensation: Adjust the game state based on estimated player latency to provide a fair experience.
- Adaptive Frame Rate: Dynamically adjust the game’s update rate based on network conditions.
- Jitter Buffer: Implement a buffer to smooth out irregular packet arrivals.
Load Balancing Strategies
Proper load balancing ensures optimal performance as player counts increase:
- Server Distribution: Utilize multiple servers across different regions to reduce latency for geographically diverse players.
- Dynamic Server Allocation: Automatically scale server resources based on player demand.
- Instance Sharding: Divide game worlds into smaller, manageable instances to distribute load.
Here’s a comparison of different optimization techniques and their impact:
Technique | Bandwidth Reduction | Latency Improvement | Scalability |
---|---|---|---|
Data Compression | High | Low | Medium |
Delta Compression | High | Medium | High |
Client-Side Prediction | Medium | High | Low |
Entity Interpolation | Low | High | Medium |
Lag Compensation | Low | High | Low |
Load Balancing | Medium | High | High |
Implementing these optimization techniques requires careful consideration of your game’s specific requirements. Start by identifying the most critical performance bottlenecks through profiling and testing. Prioritize optimizations that address these issues directly.
For example, if your game involves fast-paced action with frequent state updates, focus on implementing efficient delta compression and client-side prediction. On the other hand, if you’re developing a large-scale MMO, emphasize load balancing and instance sharding to ensure smooth performance as player counts scale.
Remember that optimization is an iterative process. Continuously monitor your game’s performance metrics and be prepared to adjust your strategies as needed. Regular testing with real players in various network conditions will provide valuable insights into the effectiveness of your optimizations.
By carefully implementing these network performance optimizations, you can significantly enhance the multiplayer experience in your Unity game, ensuring smooth gameplay even under challenging network conditions.
Implementing multiplayer game logic

Player Authentication and Connection
When implementing multiplayer game logic in Unity, player authentication and connection are crucial components. A robust authentication system ensures secure access and prevents unauthorized users from joining the game.
To implement player authentication, consider using a combination of username/password and unique player identifiers. Implement server-side validation to verify credentials and maintain a secure database of registered players. Once authenticated, assign each player a unique session token for subsequent communications.
For connection management, utilize Unity’s built-in NetworkManager or a third-party networking solution. Implement a connection handshake protocol to establish a reliable connection between the client and server. Handle connection failures gracefully, providing clear feedback to players and attempting reconnection when appropriate.
Lobby and Room Management
Effective lobby and room management are essential for organizing players and creating game instances. Implement a lobby system where players can view available rooms, create new ones, or join existing sessions.
Create a room management system that handles player joining, leaving, and room state synchronization. Consider implementing the following features:
Feature | Description |
---|---|
Room Creation | Allow players to create custom rooms with specific settings |
Room Listing | Display available rooms with relevant information (player count, game mode) |
Room Joining | Enable players to join rooms based on preferences or invitations |
Player Ready Status | Implement a ready-up system to ensure all players are prepared |
Room Chat | Add a chat feature for pre-game communication |
In-Game Communication Systems
Effective communication is vital for multiplayer games. Implement various communication systems to enhance player interaction and coordination. Consider the following options:
- Voice Chat: Integrate a voice communication system for real-time audio interaction.
- Text Chat: Implement a text-based chat system for players who prefer typing.
- Emote System: Add pre-defined emotes or quick commands for non-verbal communication.
- Ping System: Implement a ping system for players to mark locations or objects in the game world.
Ensure that these communication systems are optimized for performance and have proper moderation tools to maintain a positive gaming environment.
Syncing Game Events and Interactions
Synchronizing game events and interactions is crucial for maintaining a consistent game state across all connected clients. Implement a robust synchronization system that handles various types of game events:
- Player Actions: Sync player movements, abilities, and interactions with the game world.
- Environmental Changes: Synchronize changes to the game environment, such as destructible objects or dynamic elements.
- AI Behavior: Ensure that AI-controlled entities behave consistently across all clients.
- Loot and Item Spawns: Synchronize the spawning and collection of in-game items and resources.
Utilize Unity’s NetworkView component or custom RPC (Remote Procedure Calls) to efficiently transmit and apply these events across the network. Implement prediction and interpolation techniques to smooth out network latency and provide a seamless multiplayer experience.
Now that we’ve covered the essential aspects of implementing multiplayer game logic, the next section will focus on testing and debugging multiplayer functionality to ensure a smooth and enjoyable gaming experience for all players.
Testing and Debugging Multiplayer Functionality

Local Network Testing
Local network testing is a crucial first step in ensuring your Unity multiplayer game functions correctly. This process involves running multiple instances of your game on the same local area network (LAN) to identify and resolve basic connectivity issues.
To begin, set up a local server on one machine and connect client instances from other devices on the same network. This allows you to test fundamental multiplayer features such as player joining, synchronization, and basic interactions without the complexities of internet latency.
Key aspects to focus on during local network testing include:
- Connection establishment
- Player spawning and movement synchronization
- Object interaction and replication
- Basic game logic functionality
Here’s a comparison of local network testing methods:
Method | Pros | Cons |
---|---|---|
Multiple physical devices | Real-world scenario, Different hardware configurations | Requires multiple devices, More setup time |
Multiple instances on one PC | Quick setup, Easy debugging | May not reveal hardware-specific issues |
Virtual machines | Simulates different environments, Controlled testing | Resource-intensive, Potential performance impact |
Simulating Different Network Conditions
After successful local testing, it’s essential to simulate various network conditions to ensure your game performs well under different scenarios. Unity provides tools like the Network Simulator to emulate different network environments.
Key network conditions to simulate:
- High latency (lag)
- Packet loss
- Jitter (variable latency)
- Limited bandwidth
By testing under these conditions, you can identify potential issues such as desynchronization, rubber-banding, or unresponsive controls. This helps in implementing robust netcode that can handle less-than-ideal network situations.
Stress Testing with Multiple Clients
Stress testing is crucial to evaluate your game’s performance and stability under heavy load. This involves connecting a large number of clients simultaneously to push your server and networking code to its limits.
Key aspects of stress testing include:
- Server performance under high player counts
- Bandwidth usage scalability
- CPU and memory utilization
- Concurrency handling in game logic
Tools like Unity’s Multiplayer PlayMode Tools can help automate the process of connecting multiple clients for stress testing.
Profiling and Optimizing Network Code
Profiling your network code is essential for identifying performance bottlenecks and optimizing your multiplayer implementation. Unity’s Profiler and Network Profiler tools are invaluable for this purpose.
Key areas to focus on when profiling:
- Message size and frequency
- Serialization and deserialization efficiency
- Network update frequency
- Server-side processing time
Once bottlenecks are identified, optimize your code by implementing techniques such as:
- Data compression
- Delta compression for state updates
- Interest management to reduce unnecessary updates
- Optimizing serialization methods
By thoroughly testing, debugging, and optimizing your multiplayer functionality, you can ensure a smooth and enjoyable experience for players across various network conditions. This comprehensive approach will help you create a robust and scalable multiplayer implementation in Unity.
Also Read :
- Slithering Fun: Unleashing the Best Snake Game Unblocked – Play Anytime, Anywhere!
- 7 Unity Tools That Will Transform Your Indie Game
- How to Optimize Unity Games: A Step-by-Step Guide
- How to Publish a Game on Google Play store in 30 min
- Best Free Assets on the Unity Engine Asset Store for Indie Developers
Conclusion
Implementing multiplayer functionality in Unity requires careful consideration of various factors, from selecting the appropriate networking framework to optimizing network performance. By following the steps outlined in this guide, developers can create robust and engaging multiplayer experiences for their Unity games.

The key to successful multiplayer implementation lies in thorough planning, efficient synchronization of game states, and rigorous testing. As technology continues to evolve, staying informed about the latest networking solutions and best practices will ensure that developers can create multiplayer games that meet the expectations of modern players. Whether building a small-scale cooperative game or a large-scale competitive experience, the techniques discussed here provide a solid foundation for bringing multiplayer capabilities to Unity projects.
Frequently asked Question with Answer
Can you play Unity multiplayer?
Yes, Unity supports multiplayer functionality through various tools and services, such as Unity Multiplayer, Photon, and Mirror.
Does Unity have built in multiplayer?
Unity has built-in networking and multiplayer solutions. Unity Netcode for GameObjects is a high-level API that provides tools for building networked games.
What is the multiplayer option in Unity?
The multiplayer option in Unity allows developers to create games that can be played by multiple players simultaneously over a network. Unity provides tools and frameworks, such as UNet (deprecated) and the newer Unity Transport and Netcode for GameObjects, to facilitate the development of multiplayer functionality, including client-server architecture, matchmaking, and synchronization of game state across players.
How to make a game with multiple people in Unity?
To create a multiplayer game in Unity, you can use the Photon Unity Networking (PUN) framework. PUN provides a simple and scalable way to add multiplayer functionality to your game.
What is the best multiplayer framework for Unity?
The best multiplayer framework for Unity depends on your game type. Netcode for GameObjects is great for small projects, Photon Fusion for real-time action games, and Mirror for server-authoritative setups. Fish-Net is ideal for large-scale multiplayer.
How do I set up Unity multiplayer implementation?
Start by selecting a Unity networking setup like Netcode, Mirror, or Photon. Configure network managers, sync player movements, and implement matchmaking. Optimize latency handling for smooth gameplay.
What’s the easiest way to add multiplayer in Unity?
Using Photon PUN or Unity’s Netcode for GameObjects is the easiest way. These frameworks offer built-in tools for matchmaking, synchronization, and network handling with minimal coding.
How do I reduce lag in Unity multiplayer implementation?
Optimize network traffic with compression, interpolation, and client prediction. Use dedicated servers for better performance and Unity Relay to reduce latency.
Is Unity good for multiplayer games?
Yes! Unity supports multiple networking frameworks, real-time synchronization, and cloud-based solutions, making it a strong choice for multiplayer game development.